H5 新特性
图表
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
<style>
#canvas {
display: block;
margin: 0 auto;
border: 1px solid #333;
}
</style>
</head>
<body>
<canvas id="canvas" width="600" height="400"></canvas>
<script>
let dataset = [
{
text: "家电用器",
sales: 210,
},
{
text: "音像制品",
sales: 125,
},
{
text: "手机通讯",
sales: 98,
},
{
text: "服装服饰",
sales: 9,
},
{
text: "家居家具",
sales: 225,
},
];
let canvasEle = document.getElementById("canvas");
let ctx = canvasEle.getContext("2d");
let width = 50;
let spacerNum = dataset.length - 1;
let spacerWidth = Math.ceil(
(canvasEle.width - dataset.length * width) / spacerNum
);
ctx.fillStyle = "#900";
ctx.font = "16px Microsoft Yahei";
ctx.textAlign = "center";
dataset.forEach((item, index) => {
let x = index * (spacerWidth + width);
let y = canvasEle.height - item.sales;
ctx.fillRect(x, y, width, item.sales);
ctx.fillText(item.sales, x + Math.floor(width / 2), y - 5);
});
</script>
</body>
</html>
IP 定位
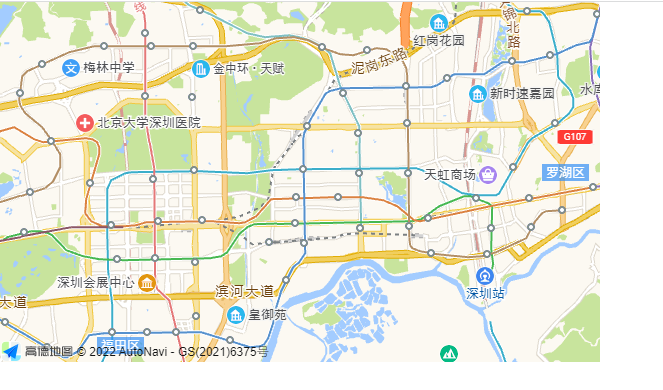
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta
name="viewport"
content="initial-scale=1.0, user-scalable=no, width=device-width"
/>
<title>根据ip定位</title>
<link
rel="stylesheet"
href="https://a.amap.com/jsapi_demos/static/demo-center/css/demo-center.css"
/>
<style type="text/css">
html,
body,
#container {
height: 360px;
width: 600px;
}
</style>
</head>
<body>
<div id="container"></div>
<div class="info">
<p id="info"></p>
</div>
<script
type="text/javascript"
src="https://webapi.amap.com/maps?v=1.4.15&key=2cc7acd5402708b09bffcd61c0b47e2a&plugin=AMap.CitySearch"
></script>
<script type="text/javascript">
var map = new AMap.Map("container", {
resizeEnable: true,
center: [114.085947, 22.547],
zoom: 13,
});
function showCityInfo() {
var citysearch = new AMap.CitySearch();
citysearch.getLocalCity(function (status, result) {
if (status === "complete" && result.info === "OK") {
if (result && result.city && result.bounds) {
var cityinfo = result.city;
var citybounds = result.bounds;
document.getElementById("info").innerHTML =
"您当前所在城市:" + cityinfo;
map.setBounds(citybounds);
}
} else {
document.getElementById("info").innerHTML = result.info;
}
});
}
showCityInfo();
</script>
</body>
</html>
canvas 高级
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
<style>
#canvas {
display: block;
margin: 0 auto;
border: 1px solid #333;
}
</style>
</head>
<body>
<canvas id="canvas"></canvas>
<script>
let canvasEle = document.getElementById("canvas");
canvasEle.width = 600;
canvasEle.height = 360;
let ctx = canvasEle.getContext("2d");
let x = 0;
let y = 0;
let xDistance = 10;
let yDistance = 10;
let i = 0;
window.setInterval(() => {
i++;
console.log(i);
ctx.clearRect(0, 0, canvasEle.width, canvasEle.height);
ctx.strokeRect(x, y, 100, 100);
x += xDistance;
y += yDistance;
if (x > canvasEle.width - 100 || x <= 0) {
xDistance = -xDistance;
}
if (y > canvasEle.height - 100 || y <= 0) {
yDistance = -yDistance;
}
}, 100);
</script>
</body>
</html>
canvas 随机触碰边界换背景色

<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
<style>
#canvas {
display: block;
margin: 0 auto;
border: 1px solid #333;
}
</style>
</head>
<body>
<canvas id="canvas"></canvas>
<p align="center">
<button onclick="play()">开始</button>
<button onclick="stop()">停止</button>
</p>
<script>
let canvasEle = document.getElementById("canvas");
canvasEle.width = 600;
canvasEle.height = 360;
let ctx = canvasEle.getContext("2d");
let x = 0;
let y = 0;
let xDistance = 2;
let yDistance = 1.5;
let i = 0;
let requestId;
let isPlaying = true;
let colors = ["#f00", "#0f0", "#00f", "#000", "#ff0"];
function animation() {
ctx.clearRect(0, 0, canvasEle.width, canvasEle.height);
ctx.fillRect(x, y, 100, 100);
x += xDistance;
y += yDistance;
if (x > canvasEle.width - 100 || x <= 0) {
xDistance = -xDistance;
ctx.fillStyle = colors[Math.floor(Math.random() * colors.length)];
}
if (y > canvasEle.height - 100 || y <= 0) {
yDistance = -yDistance;
ctx.fillStyle = colors[Math.floor(Math.random() * colors.length)];
}
requestId = window.requestAnimationFrame(animation);
}
function stop() {
if (isPlaying == true) {
window.cancelAnimationFrame(requestId);
isPlaying = false;
}
}
function play() {
if (isPlaying == false) {
animation();
isPlaying = true;
}
}
animation();
</script>
</body>
</html>
canvas 三角形 圆形
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
#canvas{
display: block;
margin: 0 auto;
border:0.5px solid #333;
}
</style>
</head>
<body>
<canvas id="canvas"></canvas>
<script>
let canvasEle = document.getElementById('canvas');
canvasEle.width = 600;
canvasEle.height = 360;
let ctx = canvasEle.getContext('2d');
ctx.beginPath();
ctx.moveTo(100,180);
ctx.lineTo(300,180);
ctx.lineTo(300,300);
ctx.lineTo(100,180);
ctx.stroke();
ctx.beginPath();
ctx.arc(400, 180, 50, 0, 2 * Math.PI);
ctx.stroke();
</script>
</body>
</html>
canvas 随机触碰边界弹跳 banner

<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
body,
p,
div,
h1,
h2,
h3,
h4,
h5,
h6 {
margin: 0;
padding: 0;
}
ul,
ol {
list-style: none;
}
#canvas {
display: block;
background: #000;
}
</style>
</head>
<body>
<canvas id="canvas"></canvas>
<script>
let canvasEle = document.getElementById('canvas');
canvasEle.width = window.innerWidth;
canvasEle.height = 320;
let ctx = canvasEle.getContext('2d');
ctx.fillStyle = '#fff';
let circles = [];
class Circle{
constructor(){
this.x = Math.floor(Math.random() * canvasEle.width);
this.y = Math.floor(Math.random() * canvasEle.height);
this.radius = Math.ceil(Math.random() * 5) + 3;
this.xSpeed = Math.random() * 2 > 1 ? Math.random() * 2 : - Math.random() * 2;
this.ySpeed = Math.random() * 2 > 1 ? Math.random() * 2 : - Math.random() * 2;
}
}
function init() {
for(n =0;n<50;n++) {
let circle = new Circle();
circles.push(circle);
ctx.beginPath();
ctx.moveTo(circle.x, circle.y);
ctx.arc(circle.x, circle.y, circle.radius, 0, 2 * Math.PI);
ctx.fill();
}
console.log(circles);
}
function animation(){
ctx.clearRect(0,0,canvasEle.width,canvasEle.height);
for(let n=0;n<50;n++){
let circle=circles[n]
circle.x += circle.xSpeed;
circle.y += circle.ySpeed;
ctx.beginPath();
ctx.moveTo(circle.x, circle.y);
ctx.arc(circle.x, circle.y, circle.radius, 0, 2 * Math.PI);
ctx.fill();
if(circle.x > canvasEle.width - circle.radius || circle.x <= 0){
circle.xSpeed = - circle.xSpeed;
}
if(circle.y > canvasEle.height - circle.radius || circle.y <= 0){
circle.ySpeed = - circle.ySpeed;
}
}
window.requestAnimationFrame(animation);
}
init();
animation();
</script>
</body>
</html>
canvas 随机触碰边界弹跳 banner2

<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
body,
p,
div,
h1,
h2,
h3,
h4,
h5,
h6 {
margin: 0;
padding: 0;
}
ul,
ol {
list-style: none;
}
#canvas {
display: block;
background: #000;
}
</style>
</head>
<body>
<div id=""></div>
<canvas id="canvas"></canvas>
<script>
let canvasEle = document.getElementById('canvas');
canvasEle.width = window.innerWidth;
canvasEle.height = 320;
let ctx = canvasEle.getContext('2d');
ctx.fillStyle = '#fff';
let circles = [];
class Circle {
constructor() {
this.x = Math.floor(Math.random() * canvasEle.width);
this.y = Math.floor(Math.random() * canvasEle.height);
this.radius = Math.ceil(Math.random() * 5) + 3;
this.xSpeed = Math.random() * 2 > 1 ? Math.random() * 2 : - Math.random() * 2;
this.ySpeed = Math.random() * 2 > 1 ? Math.random() * 2 : - Math.random() * 2;
}
}
function init() {
for (n = 0; n < 150; n++) {
let circle = new Circle();
circles.push(circle);
ctx.beginPath();
ctx.moveTo(circle.x, circle.y);
ctx.arc(circle.x, circle.y, circle.radius, 0, 2 * Math.PI);
ctx.fill();
}
console.log(circles);
}
function animation() {
ctx.clearRect(0, 0, canvasEle.width, canvasEle.height);
for (let n = 0; n <150; n++) {
ctx.fillStyle=`rgb(${255*Math.random()},${255*Math.random()},${255*Math.random()})`;
let circle = circles[n];
circle.x += circle.xSpeed;
circle.y += circle.ySpeed;
ctx.beginPath();
ctx.moveTo(circle.x, circle.y);
ctx.arc(circle.x, circle.y, circle.radius, 0, 2 * Math.PI);
ctx.fill();
if (circle.x > canvasEle.width - circle.radius || circle.x <= 0) {
circle.xSpeed = - circle.xSpeed;
}
if (circle.y > canvasEle.height - circle.radius || circle.y <= 0) {
circle.ySpeed = - circle.ySpeed;
}
}
connect();
window.requestAnimationFrame(animation);
}
function connect() {
for (let n = 0; n < 150; n++) {
for (let i = n + 1; i < 150; i++) {
let distance = Math.sqrt((circles[n].x - circles[i].x)*(circles[n].x - circles[i].x) + (circles[n].y - circles[i].y) * (circles[n].y - circles[i].y));
if(distance <= 50){
ctx.strokeStyle = `rgb(${255*Math.random()},${255*Math.random()},${255*Math.random()})`;
ctx.beginPath();
ctx.moveTo(circles[n].x,circles[n].y);
ctx.lineTo(circles[i].x,circles[i].y);
ctx.stroke();
}
}
}
}
init();
animation();
</script>
</body>
</html>